I recently had an issue where I wanted to play a movie on one of my portable devices, but the file’s audio stream is encoded in the AC3 (Dolby Digital) format. But of course, the device doesn’t support AC3, so I need to convert it to a compatible format.
Handbrake is my go-to tool for media file conversion, but it no longer supports output to XviD – specifically the AVI container. I could convert the whole file, but I was looking for a way to transcode the audio but leave the XviD video stream intact. This method is ideal because transcoding video is the most time-consuming operation of the media file conversion process. I researched further with the knowledge that Handbrake leverages FFmpeg.
Steps
- Get and install FFmpeg .
Mac OS X
Install MacPorts .
Sync the local ports tree with the global MacPorts ports repository for the latest and greatest. Run this command from the terminal:
sudo port -d selfupdate
Grab the ffmpeg
port source and compile. This will also get the required dependency packages like LAME, XviD, x264, etc. if not already installed. Run this command from the terminal:
sudo port -v install ffmpeg
Ubuntu
The FFmpeg package included in the default repository is somewhat restricted, so we will grab our FFmpeg package from the Medibuntu repository.
Refer to Compile FFmpeg on Ubuntu, Debian, or Mint for the latest information on how to install FFmpeg on Ubuntu.
Fedora
Fedora does not include the FFmpeg package in the default repositories. A third party repo will need to be added. I will use the ATrpms repository in this example.
Import the public signing key for the repository. Run the following commands from the terminal:
su -
rpm --import http://packages.atrpms.net/RPM-GPG-KEY.atrpms
Create and add the atrpms.repo
file to the /etc/yum.repos.d
directory. Run this command from the terminal:
vim /etc/yum.repos.d/atrpms.repo
Add the following to the file:
[atrpms]
name=Fedora Core $releasever - $basearch - ATrpms
baseurl=http://dl.atrpms.net/f$releasever-$basearch/atrpms/stable
gpgkey=http://ATrpms.net/RPM-GPG-KEY.atrpms
gpgcheck=1
Save the file and exit (:wq
).
We’re now ready to grab FFmpeg and the dependency packages. Run this command from the terminal:
yum install ffmpeg
- Let FFmpeg perform its magic.
File properties before the conversion:
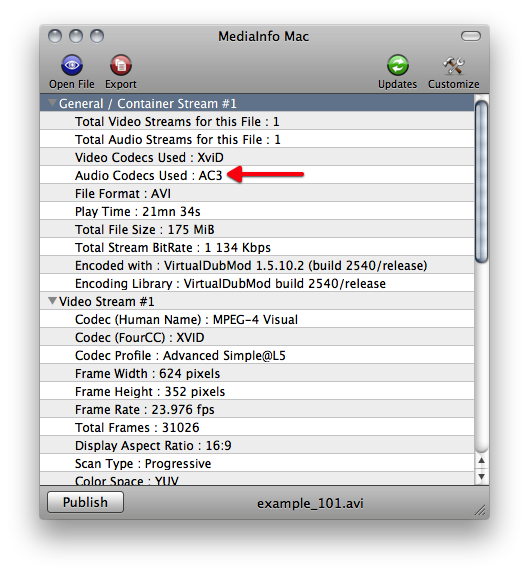
Execute the FFmpeg command with all required options and arguments. Run this command from the terminal:
ffmpeg -i "/Users/marc/Movies/example_101.avi" -vcodec copy \
-acodec libmp3lame -ab 160k -ac 2 -af volume=2.0 \
"/Users/marc/Movies/example_101_mod.avi"
The dissection of our FFmpeg command:
Option | Value | Description |
---|---|---|
-i | /Users/marc/Movies/example_101.avi | The absolute path for the input file |
-vcodec | copy | Force video codec to copy our original XviD stream |
-acodec | libmp3lame | Use the LAME MP3 encoder for the audio stream |
-ab | 160k | Audio stream bitrate |
-ac | 2 | The number of audio channels |
-af | volume=2.0 | The volume level of the audio stream |
/Users/marc/Movies/example_101_mod.avi | The absolute path for the output file |
File properties after the conversion:
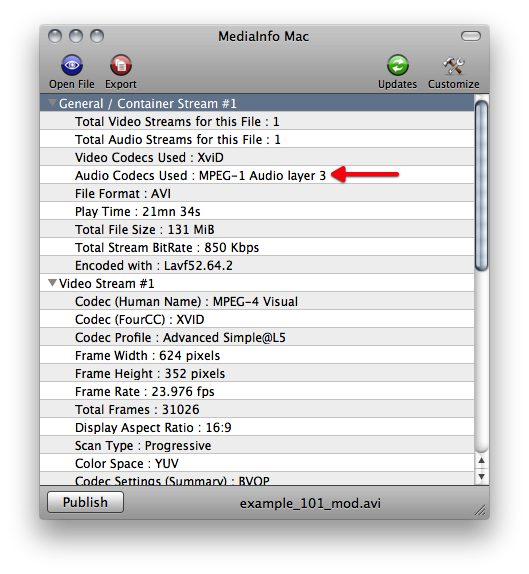
- Automate it.
Let’s take this a step further and automate the conversion process for a directory of media files. I created a shell script that takes a source directory and target directory as arguments.
#!/usr/bin/env bash
# convert-xvid-audio - Converts AC3 audio stream to MP3 for XviD files
# Set a value for the audio quality and volume
BITRATE=160 # 128,160,192,224,256,320
VOLUME='volume=2.0' # The default is 1.0 (examples: 0.5,1.5,2.0)
if [ -d "$1" ] && [ -d "$2" ]; then
find "$1" -type f -maxdepth 1 -iname '*.avi' | sed 's:.*/::' | while read FN
do
ffmpeg -i "$1"/"$FN" -vcodec copy -acodec libmp3lame \
-ab ${BITRATE}k -ac 2 -af $VOLUME \
"$2"/"${FN%.*}_mod.avi" < /dev/null;
done
exit 0
else
echo "usage: $(basename $0) source_directory target_directory" >&2
exit 1
fi
Save the script as convert-xvid-audio.sh
.
Give the script the execute permission. Run this command from the terminal:
chmod u+x convert-xvid-audio.sh
The following command will process the original XviD files in FolderY and output the modified files to FolderZ after the conversion:
./convert-xvid-audio.sh ~/Movies/FolderY ~/Movies/FolderZ